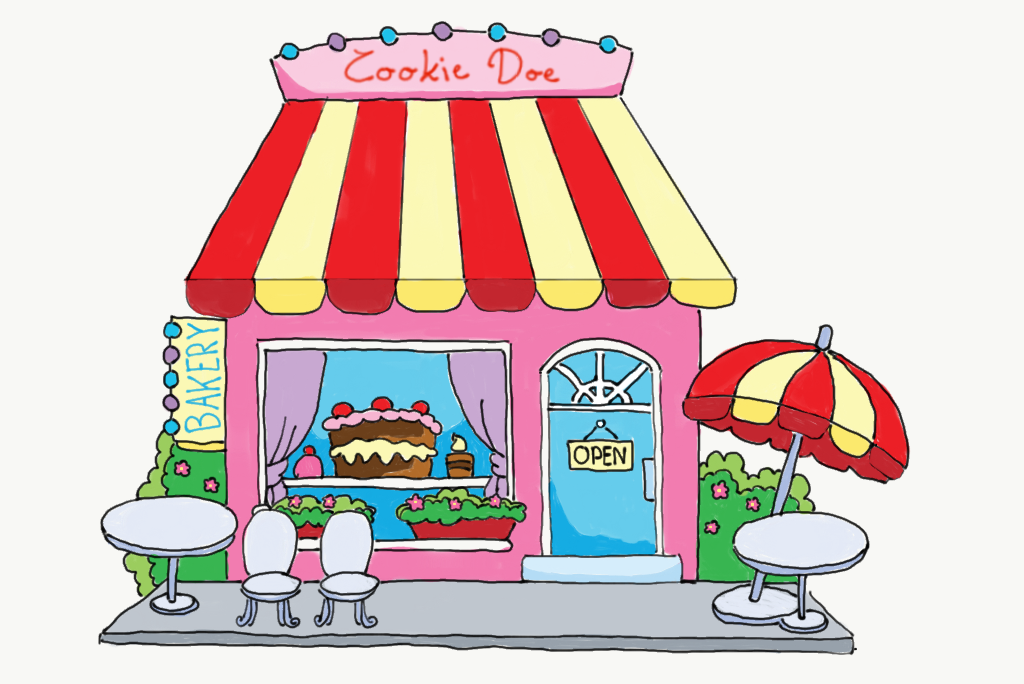
On our journey to understanding the SwiftUI framework, we will be solving some problems in the great and splendid Mangoville, a small town that doesn’t have access to Xcode. I already got tons of requests from Mangopolitans!
We just got a letter from Miss Cookie Doe, a 312-year-old baker who still loves her job! It says:
Dear Swift Kid,
I need your help. People around here have become so busy with their work that they don’t even have time to come by for a little croissant! I’ll make you a deal: if you make me an app that lets my customers order from their homes, I’ll give you free cookies for an entire year!
Sincerely,
Cookie Doe
P.S. Everything I’m selling costs $1.
We must help her! I guess we’ll need steppers and pickers. No… I’m not talking about the steppers you play with at parks or the cherry pickers you see at some construction sites. I’m talking about the SwiftUI Picker and Stepper views! I certainly hope you got it because I am moving on… just kidding! You’ll probably understand it better with a drawing:
I hope that helped. However, we still need to create our app. Start by making a new project by clicking on File > New > Project.
Before adding the picker, go ahead and add these two variables right under “struct ContentView: View {”:
@State private var selectedSnackIndex = 0 var snacks = [“croissant”, “cookie”, “brownie”, “apple pie”, “gingerbread”]
By the way, Miss Cookie Doe makes quite the apple pie. You should try it sometime! Anyways, it’s time to add the picker. Simply click that little plus icon, then drag the picker into your struct’s body.
Replace the body’s content with this code:
Picker(selection: $selectedSnackIndex, label: Text(“”)) { ForEach(0 ..< snacks.count) { Text(self.snacks[$0]) } }
You see that little dollar sign before our @State variable? That dollar sign might be small, but it makes a big difference. In fact, it gives the Picker view the right to edit the selectedSnackIndex variable. Most importantly, do not forget its awesome and complicated name… *drumrolls* a Binding! Anyways, this code builds a Picker view that lets you choose which snack you want; it goes through the snacks array and takes care of showing every snack.
Now we need to add a Stepper view, which takes care of helping the customer choose the number of snacks they want. Before adding the Stepper view, add that variable to your struct, along with the other variables:
@State private var numberOfSnacks = 0
Then, simply paste that Stepper view code right after your Picker view:
Stepper("How many would you like?", onIncrement: { self.numberOfSnacks += 1 }, onDecrement: { self.numberOfSnacks -= 1 } )
What’s that? You just got an error? Well that’s because we need a VStack. You see, a VStack takes care of stacking your views in a vertical orientation. Go ahead and wrap everything inside the body in a VStack. Once that’s done, take a moment and look at your progress in the SwiftUI Previews window. We still need to add two Text views: one that takes care of asking customers what they want and one that takes care of showing the total price. Add this to the beginning of the VStack:
Text("What would you like to eat?")
Now, all that’s left is for us to add the second Text view and a little padding to the entire VStack. Add that next to our VStack’s closing brace:
.padding()
Next, add this code at the end of the VStack:
Text("That will be \(numberOfSnacks) \(snacks[selectedSnackIndex])(s) for only $\(numberOfSnacks). Thank you very much!")
Yay, we’re done! Just make sure your file looks like this and that your app works just like in the gif below. After that, you’ll be good to go:
import SwiftUI struct ContentView: View { @State private var selectedSnackIndex = 0 @State private var numberOfSnacks = 0 var snacks = ["croissant", "cookie", "fudge", "apple pie", "gingerbread"] var body: some View { VStack { Text("What would you like to eat?") Picker(selection: $selectedSnackIndex, label: Text("")) { ForEach(0 ..< snacks.count) { Text(self.snacks[$0]) } } Stepper("How many would you like?", onIncrement: { self.numberOfSnacks += 1 }, onDecrement: { self.numberOfSnacks -= 1 } ) Text("That will be \(numberOfSnacks) \(snacks[selectedSnackIndex])(s) for only $\(numberOfSnacks). Thank you very much!") } .padding() } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } }
Awesome! We just finished building Miss Cookie Doe’s great app, and let’s just say that she’ll be giving away a lot of free cookies this year…
Update: If you want to test out the final result, you can download the project from my GitHub page here.
1 Comment
Wow man, this was really helpful! Keep up the good work.
Sincerely from your mate,
Oliver